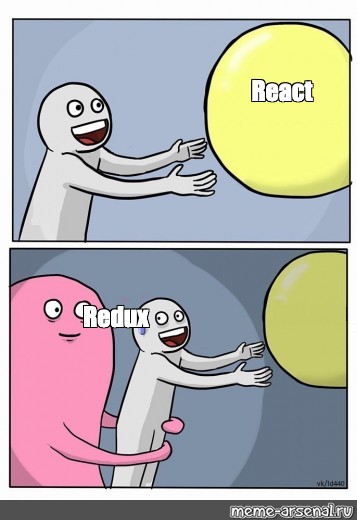
Hey, football fans! Today we’re gonna talk about React Redux, which is a state management library for React.
If you’re a Liverpool FC fan like me, you’ll be stoked to hear about some examples we can use to explain this concept. So, let’s get started!
React Redux
Redux is a library that helps you manage application state in a predictable and organized way.
It works by creating a single store that holds all the data for your application.
React Redux is a binding library that lets you use Redux with React.
Here are the steps you need to take to use React Redux in your application:
Install React Redux
You can install React Redux using npm by running the command “npm install react-redux“.
Set up a Redux store
To set up a Redux store, you need to create a store with some initial state and a reducer function that takes the current state and an action and returns a new state.
Here’s an example
import { createStore } from 'redux'; // Define an initial state for the store const initialState = { goals: 0, assists: 0, }; // Define a reducer function that updates the state based on actions function reducer(state = initialState, action) { switch (action.type) { case 'ADD_GOAL': // If the action type is "ADD_GOAL", update the "goals" property of the state by incrementing it by 1 return { ...state, goals: state.goals + 1 }; case 'ADD_ASSIST': // If the action type is "ADD_ASSIST", update the "assists" property of the state by incrementing it by 1 return { ...state, assists: state.assists + 1 }; default: // If the action type doesn't match any of the cases above, return the current state return state; } } // Create a store using the reducer function const store = createStore(reducer);
This code sets up a store with some initial state and a reducer function that handles two actions: “ADD_GOAL” and “ADD_ASSIST“.
When an action is dispatched, the reducer returns a new state with the appropriate changes.
Connect your components to the store
To connect your components to the store, you need to use the “connect” function from React Redux.
Here’s an example
import { connect } from 'react-redux'; // Define a functional component to display player statistics function PlayerStats(props) { return ( <div> <p>Goals: {props.goals}</p> <p>Assists: {props.assists}</p> </div> ); } // Define a function that maps the state to the component's props function mapStateToProps(state) { return { goals: state.goals, // The "goals" property of the state will be mapped to the "goals" prop of the component assists: state.assists, // The "assists" property of the state will be mapped to the "assists" prop of the component }; } // Connect the component to the Redux store and map the state to props using the "mapStateToProps" function export default connect(mapStateToProps)(PlayerStats);
This code connects the “PlayerStats” component to the store by mapping the state to props using the “mapStateToProps” function.
The “connect” function then returns a new component that has access to the store.
Now that we’ve gone through the steps, let’s take a look at some Liverpool FC examples.
Let’s say you’re building an app that displays player stats for Liverpool’s top goal scorers.
Here’s how you could use React Redux to manage the state
import { createStore } from 'redux'; import { connect } from 'react-redux'; // Define the initial state of the application const initialState = { players: [ { name: 'Mohamed Salah', goals: 19 }, { name: 'Sadio Mane', goals: 13 }, { name: 'Roberto Firmino', goals: 8 }, ], }; // Define a reducer function that handles state updates function reducer(state = initialState, action) { switch (action.type) { // If the action type is ADD_GOAL, update the goals for the player with the given name case 'ADD_GOAL': const players = state.players.map((player) => { if (player.name === action.payload.playerName) { return { ...player, goals: player.goals + 1 }; } return player; }); // Return a new state object with the updated players array return { ...state, players }; // If the action type is not recognized, return the current state default: return state; } } // Create a Redux store with the reducer function and the initial state const store = createStore(reducer); // Define a presentational component that displays player stats and a button to add goals function PlayerStats(props) { return ( <div> {/* Map over the players in the Redux store and display their stats */} {props.players.map((player) => ( <div key={player.name}> <h2>{player.name}</h2> <p>Goals: {player.goals}</p> {/* When the button is clicked, dispatch an action to add a goal for the player */} <button onClick={() => props.addGoal(player.name)}>Add Goal</button> </div> ))} </div> ); } // Define a function that maps the state of the Redux store to props for the presentational component function mapStateToProps(state) { return { players: state.players, }; } // Define a function that maps dispatch actions to props for the presentational component function mapDispatchToProps(dispatch) { return { addGoal: (playerName) => dispatch({ type: 'ADD_GOAL', payload: { playerName } }), }; } // Connect the presentational component to the Redux store using the connect() function const ConnectedPlayerStats = connect(mapStateToProps, mapDispatchToProps)(PlayerStats); // Export the connected component as the default export of the module export default ConnectedPlayerStats;
This code sets up a store with an initial state that includes an array of players and their goals.
The reducer function handles the “ADD_GOAL” action by finding the player with the matching name and incrementing their goals.
The “mapStateToProps” function maps the state to props, and the “mapDispatchToProps” function maps dispatch functions to props.
The “connect” function then returns a new component that has access to the store.
In the “PlayerStats” component, we map over the players and display their stats along with a button that dispatches the “ADD_GOAL” action when clicked.
When the button is clicked, the reducer updates the state, and the component re-renders with the new data.

Conclusion
So, there you have it, folks. That’s how you can use React Redux to manage state in your application, with examples tailored to Liverpool FC.
Give it a try and see how it can simplify your application’s data management.
Peace out!