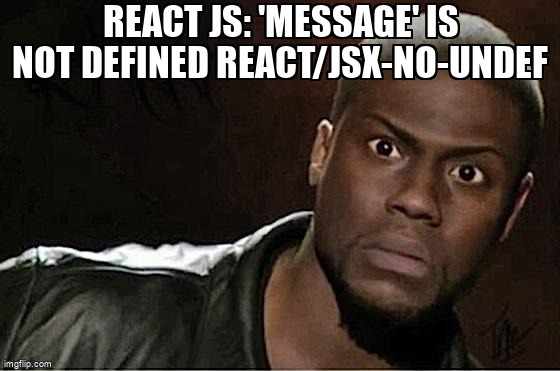
Yo, what’s up football fans? If you’re looking to get started with React, then JSX is a must-know. So, let’s dive in!
React JSX
First off, what is JSX? It’s a syntax extension to JavaScript that allows you to write HTML-like code in your JavaScript files.
This makes it easy to describe what your UI should look like and makes your code more readable.
To get started with JSX, you’ll need to install React and set up a new project.
Once you’ve done that, you can start writing JSX in your components.
Let’s take a look at an example:
import React from 'react'; function App() { return ( <div className="app"> <h1>Liverpool FC News</h1> <p>Get the latest news about Liverpool FC!</p> </div> ); } export default App;
In this code, we’re defining a new component called “App“.
Inside the component, we’re using JSX to create a div element with a class name of “app“.
We’re also creating an h1 element and a p element inside the div. Easy, right?
Now, let’s take a look at another example.
Let’s say we want to display the latest news article from Liverpool FC’s website.
We can use the official Liverpool FC API to get the data we need.
Here’s an example of how to use the API with JSX:
import React, { useState, useEffect } from 'react'; import axios from 'axios'; function App() { const [article, setArticle] = useState({}); useEffect(() => { axios.get('https://www.liverpoolfc.com/api/v1/articles/latest').then(response => { setArticle(response.data.article); }); }, []); return ( <div className="app"> <h1>{article.title}</h1> <p>{article.summary}</p> </div> ); } export default App;
In this code, we’re using React’s useState and useEffect hooks to fetch the latest news article from the Liverpool FC API and store it in state.
We’re then using JSX to display the article’s title and summary.

Conclusion
So, there you have it, folks. That’s a quick introduction to JSX in React.
If you’re a Liverpool fan, you can use JSX to create some amazing UIs for your Liverpool-themed apps.
And if you’re not a Liverpool fan, well, you can still use JSX to create some awesome apps.
Peace out!