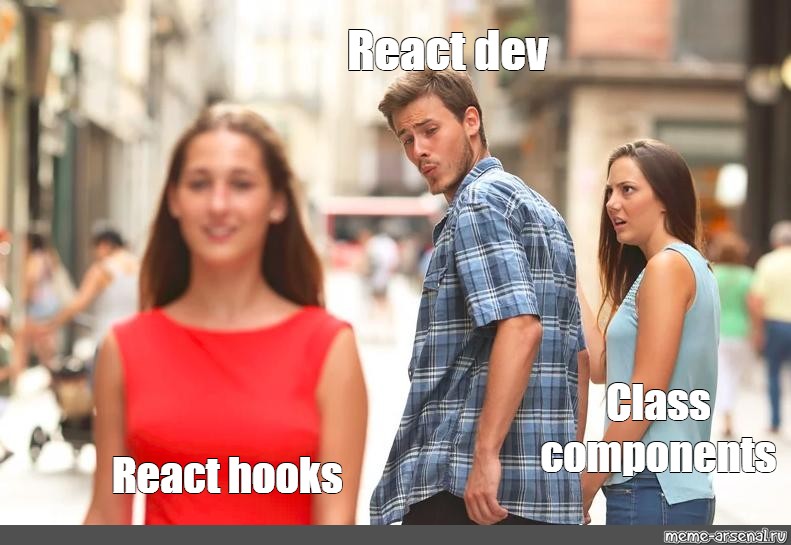
React Hooks
Hey, what’s up football fans! Today we’re gonna talk about React Hooks, which are some of the most popular features in React.
If you’re a Liverpool FC fan like me, you’ll wanna stick around for some dope examples.
First off, what are React Hooks? Hooks are functions that let you “hook into” React state and lifecycle methods from function components.
They were introduced in React 16.8 and have since become a staple in React development.
Now, let’s dive into some examples.
Let’s say you’re building an app that displays Liverpool FC’s starting lineup for a match.
You can use the useState hook to store the lineup data
import React, { useState } from 'react'; // Define a function component called StartingLineup function StartingLineup() { const [lineup, setLineup] = useState([]); // Make an API call to get the starting lineup data when the component mounts useEffect(() => { fetch('https://www.liverpoolfc.com/api/v1/starting-lineup') .then(response => response.json()) .then(data => setLineup(data)); }, []); // Render the starting lineup as a list of players return ( <div> <h2>Starting Lineup</h2> <ul> {lineup.map(player => ( <li key={player.id}>{player.name}</li> ))} </ul> </div> ); } // Export the StartingLineup component as the default export of the module export default StartingLineup;
In this code, we have used the useState hook to create a lineup state variable and a setLineup function to update the lineup data.
We then use the useEffect hook to make an API call to get the starting lineup data and update the lineup state variable when the component mounts.
Now let’s take a look at another example.
Let’s say you’re building a form that allows users to submit their predictions for Liverpool FC’s next match.
You can use the useForm hook from the React Hook Form library to handle form validation and submission:
import React from 'react'; import { useForm } from 'react-hook-form'; // Define a function component called PredictionsForm function PredictionsForm() { const { register, handleSubmit, errors } = useForm(); // Handle the form submission function onSubmit(data) { // Make an API call to submit the prediction data console.log(data); } // Render a form with fields for entering a predicted score and scorer return ( <form onSubmit={handleSubmit(onSubmit)}> <label htmlFor="score">Predicted score:</label> <input id="score" type="text" name="score" ref={register({ required: true, // The score field is required pattern: /^\d{1,2}-\d{1,2}$/, // The score should be in the format "x-y" })} /> {errors.score && <p>Please enter a valid score (e.g. 2-1)</p>} <label htmlFor="scorer">Predicted scorer:</label> <input id="scorer" type="text" name="scorer" ref={register({ required: true })} // The scorer field is required /> {errors.scorer && <p>Please enter a predicted scorer</p>} <button type="submit">Submit</button> </form> ); } // Export the PredictionsForm component as the default export of the module export default PredictionsForm;
In this code, we have used the useForm hook from the React Hook Form library to handle form validation and submission.
We use the register function to register form inputs and their validation rules, and we use the handleSubmit function to handle form submission.

Conclusion
So, there you have it, folks. React Hooks are a powerful and easy-to-use feature that can make your React development experience much more enjoyable.
Whether you’re a Liverpool fan or not, give them a try in your next project and see how they can simplify your code.
Peace out!