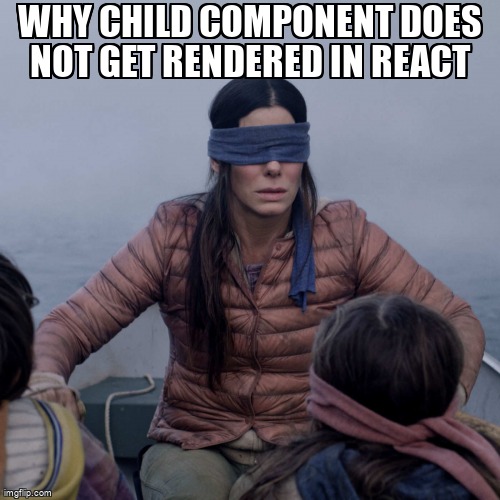
Hey there, Liverpool fans! If you’re interested in building a website or web application, you may have heard of React, a popular JavaScript library for building user interfaces.
Here we’re gonna talk about two important concepts in React: components and state.
Components
In React, a component is a reusable piece of code that encapsulates a part of a user interface.
Think of it as a building block for your web app.
In our example, we’ll create a component for displaying Liverpool FC fixtures.
Here’s what the code might look like:
import React from 'react'; function Fixtures(props) { return ( <div> <h1>{props.title}</h1> <ul> {props.fixtures.map((fixture) => ( <li key={fixture.id}> {fixture.date} - {fixture.opponent} </li> ))} </ul> </div> ); } export default Fixtures;
In this example, we define a function component called Fixtures.
The component takes in a props object as an argument, which contains the data we want to display.
We use the props to render a title and a list of fixtures.
Props
Props (short for properties) are a way of passing data from a parent component to a child component.
In our example, we pass the title and fixtures data as props to the Fixtures component.
Here’s how we might use the component in another component:
import React from 'react'; import Fixtures from './Fixtures'; function App() { const fixturesData = [ { id: 1, date: '1 Jan', opponent: 'Arsenal' }, { id: 2, date: '10 Jan', opponent: 'Chelsea' }, { id: 3, date: '15 Jan', opponent: 'Manchester United' }, ]; return ( <div> <h1>Liverpool Fixtures</h1> <Fixtures title="Upcoming Fixtures" fixtures={fixturesData} /> </div> ); } export default App;
In this example, we create an App component that renders the Fixtures component.
We pass the fixturesData array as a prop called fixtures and the string “Upcoming Fixtures” as a prop called title.
State
State is a way of storing and managing data within a component.
It allows you to update the data and trigger a re-render of the component.
In our example, we’ll use state to keep track of the number of goals Liverpool has scored this season.
import React, { useState } from 'react'; function Goals(props) { const [goals, setGoals] = useState(0); function handleClick() { setGoals(goals + 1); } return ( <div> <h1>{props.title}</h1> <p>Goals: {goals}</p> <button onClick={handleClick}>Score!</button> </div> ); } export default Goals;
In this example, we define a function component called Goals.
We use the useState hook to create a state variable called goals, which starts at 0.
We also define a function called handleClick that updates the goals state when a button is clicked.
We use the goals state to render the number of goals scored.

Conclusion
Components, props, and state are essential concepts in React.
With these tools, you can create reusable and dynamic user interfaces for your web app.
And for Liverpool fans, you can use React to build your own Liverpool-themed web app!
Whether you’re a beginner or an experienced developer, mastering these
Peace out!