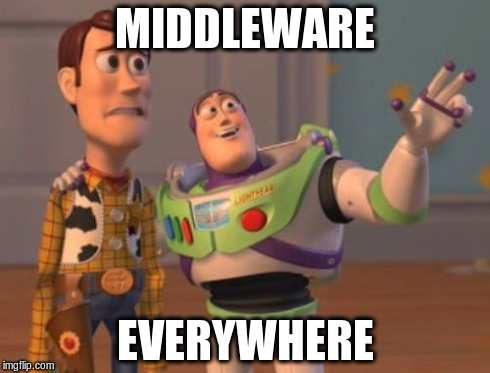
Node.js with Express.js
Hey football fans, are you interested in learning about Node.js with Express.js?
These technologies can help you build powerful web applications and APIs.
Here, we’ll explore what Node.js and Express.js are.
Node.js is an open-source, cross-platform JavaScript runtime environment that allows developers to run JavaScript code on the server-side.
It’s built on Chrome’s V8 JavaScript engine, which makes it incredibly fast and efficient.
Express.js, on the other hand, is a minimalist web framework for Node.js.
It provides a simple and flexible way to build web applications and APIs.
Now, let’s take a look at some examples.
First, let’s create a simple Express.js application that displays Liverpool FC’s current squad on the homepage.
Here’s the code:
const express = require('express'); const app = express(); const squad = [ { name: 'Alisson Becker', position: 'GK' }, { name: 'Trent Alexander-Arnold', position: 'RB' }, { name: 'Virgil van Dijk', position: 'CB' }, { name: 'Joel Matip', position: 'CB' }, { name: 'Andy Robertson', position: 'LB' }, { name: 'Fabinho', position: 'CDM' }, { name: 'Jordan Henderson', position: 'CM' }, { name: 'Thiago Alcantara', position: 'CM' }, { name: 'Sadio Mane', position: 'LW' }, { name: 'Mohamed Salah', position: 'RW' }, { name: 'Roberto Firmino', position: 'CF' } ]; app.get('/', (req, res) => { res.send(` <h1>Liverpool FC Squad</h1> <ul> ${squad.map(player => `<li>${player.name} - ${player.position}</li>`).join('')} </ul> `); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this code, we require the express module and create an instance of the app object.
We define a squad array that contains an object for each player, with their name and position.
We then define a GET route for the homepage (‘/‘) that sends an HTML response containing a list of the players in the squad.
Next, let’s create an API endpoint that returns a list of Liverpool FC’s recent fixtures. Here’s the code:
const express = require('express'); const app = express(); const fixtures = [ { homeTeam: 'Liverpool', awayTeam: 'Everton', date: '2022-03-05', result: '2-0' }, { homeTeam: 'Brentford', awayTeam: 'Liverpool', date: '2022-03-12', result: '1-2' }, { homeTeam: 'Liverpool', awayTeam: 'Manchester United', date: '2022-03-19', result: '3-1' } ]; app.get('/fixtures', (req, res) => { res.json(fixtures); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this code, we define a fixtures array that contains an object for each fixture, with the home team, away team, date, and result. We then define a `GET route for the /fixtures endpoint that sends a JSON response containing the fixtures list.
Finally, let’s add some middleware to our Express.js application that logs the current date and time for each request.
Here’s the updated code:
const express = require('express'); const app = express(); const squad = [ { name: 'Alisson Becker', position: 'GK' }, { name: 'Trent Alexander-Arnold', position: 'RB' }, { name: 'Virgil van Dijk', position: 'CB' }, { name: 'Joel Matip', position: 'CB' }, { name: 'Andy Robertson', position: 'LB' }, { name: 'Fabinho', position: 'CDM' }, { name: 'Jordan Henderson', position: 'CM' }, { name: 'Thiago Alcantara', position: 'CM' }, { name: 'Sadio Mane', position: 'LW' }, { name: 'Mohamed Salah', position: 'RW' }, { name: 'Roberto Firmino', position: 'CF' } ]; const fixtures = [ { homeTeam: 'Liverpool', awayTeam: 'Everton', date: '2022-03-05', result: '2-0' }, { homeTeam: 'Brentford', awayTeam: 'Liverpool', date: '2022-03-12', result: '1-2' }, { homeTeam: 'Liverpool', awayTeam: 'Manchester United', date: '2022-03-19', result: '3-1' } ]; const logger = (req, res, next) => { console.log(`[${new Date().toLocaleString()}] ${req.method} ${req.url}`); next(); }; app.use(logger); app.get('/', (req, res) => { res.send(` <h1>Liverpool FC Squad</h1> <ul> ${squad.map(player => `<li>${player.name} - ${player.position}</li>`).join('')} </ul> `); }); app.get('/fixtures', (req, res) => { res.json(fixtures); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this code, we define a logger middleware function that logs the current date and time, request method, and URL for each request.
We then use the app.use() method to apply this middleware to all routes.
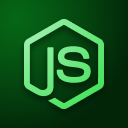
Conclusion
With these examples, you can see how Node.js and Express.js can be used to build web applications and APIs.
Whether you’re a die-hard Liverpool FC fan or just interested in learning about these technologies, Node.js and Express.js offer a powerful and flexible way to build server-side applications.
Peace out!