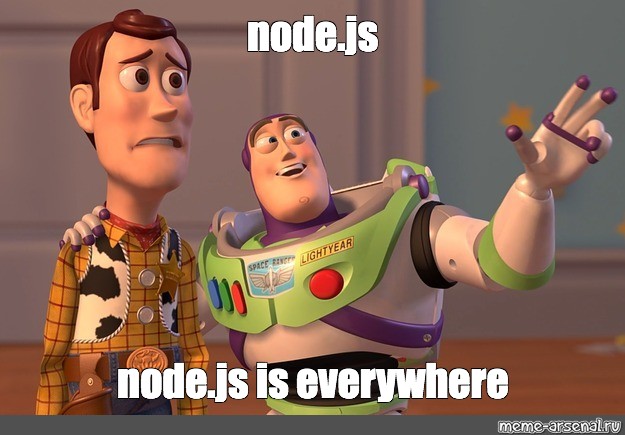
Yo, what’s up, football peeps? Are you ready to kick off your Node.js journey?
If you are, then let’s dive into the basics of Node.js.
Node JS
First things first, Node.js is a JavaScript runtime built on the V8 JavaScript engine.
It allows you to run JavaScript code on the server-side, instead of just the client-side.
Node.js uses an event-driven, non-blocking I/O model, which makes it lightweight and efficient.
Let’s break that down for ya.
Node.js lets you run your code on the server, which means you can build back-end applications and APIs with JavaScript.
It uses an event-driven model, which means that your code can respond to events (like user input or a request from a client) without blocking the rest of the application.
One of the cool things about Node.js is that it comes with a bunch of built-in modules, such as the http module.
This module lets you create a simple web server with just a few lines of code. Here’s an example:
const http = require('http'); const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello, Liverpool Fans!\n'); }); server.listen(3000, () => { console.log('Server running on port 3000'); });
This code creates a web server that responds with the message “Hello, Liverpool Fans!” when a client makes a request.
The http.createServer() method takes a callback function that is called every time a request is made to the server.
The callback function takes two arguments, req (short for “request”) and res (short for “response”).
The req object contains data about the client’s request,
and the res object is used to send a response back to the client.
Another cool thing about Node.js is that it has a package manager called npm (short for “Node Package Manager“).
npm lets you install and manage third-party modules that you can use in your Node.js applications.
For example, let’s say you wanted to use the express module to create a more robust web application.
You could install it using npm like this:
npm install express
const express = require('express'); const app = express(); app.get('/', (req, res) => { res.send('Hello, Liverpool fans!'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
This code creates an Express.js application that responds with the message “Hello, Liverpool fans!” when a client makes a request to the root URL (/)
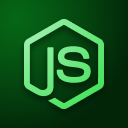
Conclusion
So, there you have it, folks! That’s a quick introduction to the basics of Node.js
Now it’s time to hit the pitch and start building some awesome back-end applications with Node.js!
Peace out!