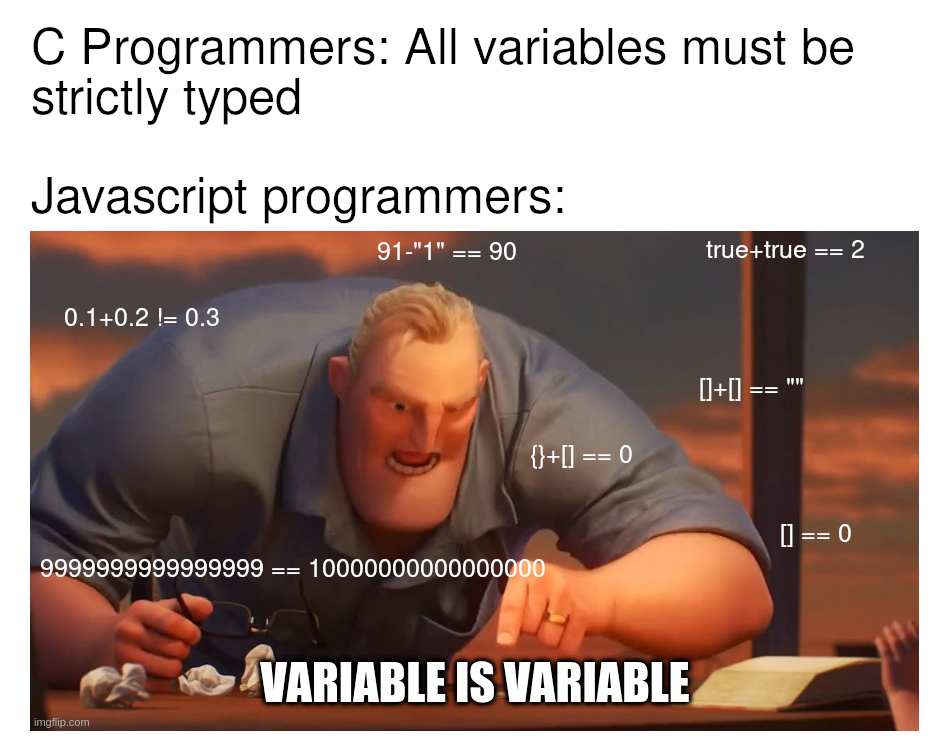
JavaSript
Yo, what’s good football fans! Today, we’re gonna talk about JavaScript, the MVP of web development.
JavaScript is like a Lionel Messi, it can dribble circles around any challenge and score some amazing goals.
Let’s dive into some JavaScript examples using football terminology.
Variables and Data Types:
In football, each player has their own unique skill set and position, just like variables in JavaScript.
A variable is like a player, and it has a name, position, and value. For example:
var striker = "Lionel Messi"; var position = "Forward"; var goals = 700;
In this example, we have three variables: striker, position, and goals.
striker has a value of “Lionel Messi”,
position has a value of “Forward”,
and goals has a value of 700.
These variables have different data types, just like football players have different skills and abilities.
Data types in JavaScript include strings, numbers, booleans, null, undefined, and objects. For example:
var club = "Barcelona"; var founded = 1899; var hasWonChampionsLeague = true; var squad = null; var coach; console.log(typeof club); // string console.log(typeof founded); // number console.log(typeof hasWonChampionsLeague); // boolean console.log(typeof squad); // object console.log(typeof coach); // undefined
In this example, we have several variables with different data types. club is a string, founded is a number, hasWonChampionsLeague is a boolean, squad is null, and coach is undefined.
We use the typeof operator to check the data type of each variable.
Operators:
In football, players use different techniques to gain an advantage, just like operators in JavaScript.
JavaScript includes several types of operators, such as arithmetic, comparison, logical, and assignment operators.
Let’s look at some examples:
var goals = 700; var assists = 300; console.log(goals + assists); // 1000 console.log(goals > assists); // true console.log(goals == assists); // false var club = "Barcelona"; club += " FC"; console.log(club); // "Barcelona FC"
In this example, we have two variables, goals and assists.
We use the + operator to add the values of goals and assists.
We use the > operator to compare the values of goals and assists,
The == operator to check if they’re equal.
We also use the += operator to concatenate the string ” FC” to the club variable.
JavaScript also includes other types of operators, such as
Logical operators (&&, ||, !),
Bitwise operators (&, |, ^, ~, <<, >>, >>>)
Conditional operators (?, :).
Conclusion
JavaScript variables and data types, as well as operators, are important concepts to understand in order to develop powerful web applications.
Just like in football, each player has their own unique skills and techniques, and by learning the fundamentals of JavaScript, you can use these tools to create amazing web experiences.
Peace out!