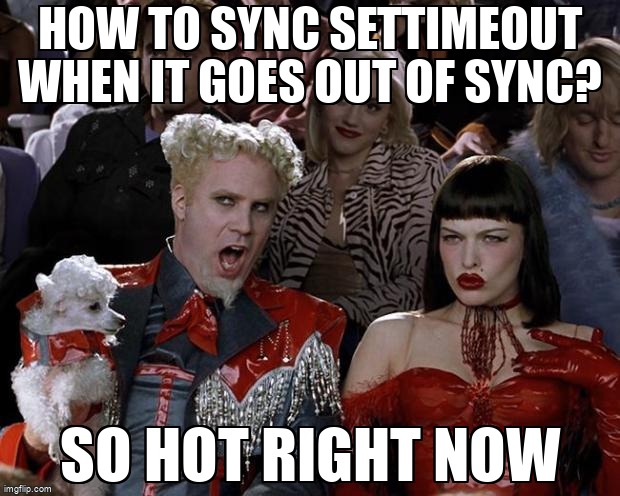
Hey football fans, today we’re going to talk about two important concepts in JavaScript: Event Loop and Modules.
Just like in football, where players must coordinate their movements and actions, in JavaScript, events must be handled in the right order
and Modules must be organized to work together seamlessly. So, let’s dive in!
Event Loop
In football, the game is constantly in motion, with players moving around the field and making plays.
Similarly, in JavaScript, events are constantly happening, such as mouse clicks, key presses, and network requests.
The event loop is a mechanism that allows JavaScript to handle these events in a non-blocking way, while still maintaining the order of execution.
To understand the event loop, let’s look at an example:
console.log("1"); setTimeout(function() { console.log("2"); }, 1000); console.log("3");
In this example, we have three console.log statements, with a setTimeout function in the middle.
The setTimeout function is a built-in JavaScript function that allows you to schedule a function to run after a specified amount of time.
In this case, we’re scheduling a function that logs “2” to the console, after a delay of one second.
Now, if we run this code, we might expect to see the output “1”, “3”, “2”.
However, due to the event loop, the output will actually be “1”, “3”, “2” after one second.
Here’s why:
The first console.log statement logs “1” to the console.
The setTimeout function schedules the function that logs “2” to the console to run after one second.
The third console.log statement logs “3” to the console.
After one second, the function scheduled by setTimeout is executed, logging “2” to the console.
In other words, the event loop allows JavaScript to handle asynchronous events, like the delay in the setTimeout function, without blocking the execution of other code.
Modules
In football, players have specific roles and responsibilities, and they work together to achieve a common goal. Similarly, in JavaScript, modules allow you to break up your code into smaller, more manageable pieces, each with its own specific purpose.
To understand modules, let’s look at an example:
// module.js export function greet(name) { console.log("Hello, " + name + "!"); } // main.js import { greet } from "./module.js"; greet("Lionel Messi");
In this example, we have two files: module.js and main.js.
In module.js, we define a function called greet that logs a greeting to the console.
We also use the export keyword to make the greet function available to other modules.
In main.js, we use the import keyword to import the greet function from module.js.
We then call the greet function and pass in “Lionel Messi” as the name parameter.
Modules are a powerful way to organize your code and keep it modular and reusable.
They allow you to break your code into smaller, more manageable pieces, each with its own specific purpose.
Conclusion
In conclusion, the event loop and modules are two important concepts in JavaScript that allow you to write efficient, maintainable, and scalable code.
Just like in football, where coordination and teamwork are key to success, in JavaScript, understanding these concepts and how they work together can help you build better and more powerful applications.
Peace out!