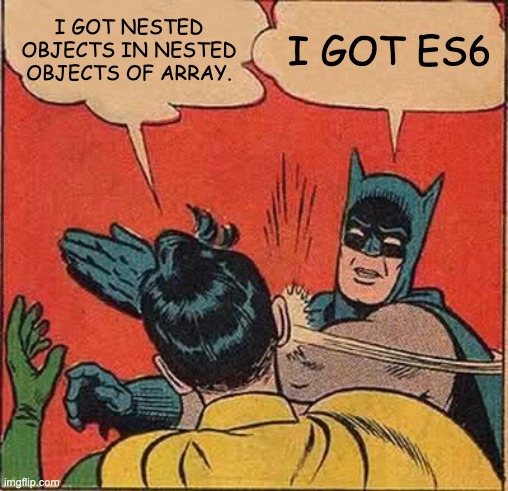
Hey football fans, are you ready to take your JavaScript game to the next level?
ES6+ (ECMAScript 6) is here with some new features that will help you score some major goals in your coding projects.
Let’s kick things off with some examples using football terminology:
Arrow Functions
Arrow functions are like a quick pass in football – they get the job done with minimal effort.
They are a shorter way to write functions in JavaScript. Here’s an example:
const getTeamName = (team) => ${team} Football Club;
In this example, we use an arrow function to return a string that includes the team name.
Template Literals
Template literals are like a customized jersey – they allow you to insert variables into strings easily.
They are a new way to write strings in JavaScript. Here’s an example:
const teamName = 'Liverpool'; const message = Welcome to ${teamName} Football Club!;
In this example, we use template literals to create a message that includes the team name.
Destructuring
Destructuring is like breaking down a defense.
It allows you to extract values from objects or arrays easily. Here’s an example:
const player = { name: 'Mohamed Salah', position: 'Forward', goals: 20 }; const { name, position, goals } = player;
In this example, we use destructuring to create variables that contain the values from the player object.
Spread Operator
The spread operator is like spreading the ball across the field.
It allows you to expand arrays or objects easily. Here’s an example:
const firstHalf = ['Liverpool', '2-0', 'Chelsea']; const secondHalf = ['Chelsea', '1-3', 'Liverpool']; const matchResult = [...firstHalf, ...secondHalf];
In this example, we use the spread operator to combine the firstHalf and secondHalf arrays into a single array called matchResult.
Conclusion
ES6+ also includes other features such as classes, let and const, default parameters, and more.
By using these features, you can improve the readability and maintainability of your code.
So, next time you’re writing JavaScript code, just think of it as a football match – with the help of ES6+, you can score some major goals and take your coding game to the next level.
Peace out!