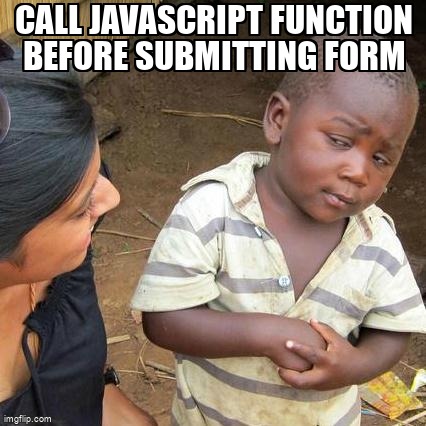
Yo, what’s up football fans! Today, we’re gonna talk about JavaScript control flow and functions, the game changers of web development.
These concepts are like tactics and strategies used by coaches to control the game and score goals. Let’s dive into some JavaScript examples using football terminology.
Control Flow
In football, coaches use tactics to control the flow of the game and outmaneuver the opponents, just like control flow statements in JavaScript.
A control flow statement is a block of code that determines the order in which statements are executed.
The most common control flow statements in JavaScript are if/else statements, switch statements, and loops.
If/Else Statements
If/else statements are like tactics that allow you to execute different code based on a condition. For example:
var score = 1; if (score == 0) { console.log("We're losing!"); } else if (score == 1) { console.log("We're tied!"); } else { console.log("We're winning!"); }
In this example, we have a variable called score that has a value of 1.
We used an if/else statement to check the value of score and log a message to the console checking if we’re winning, losing, or tied.
Switch Statements
Switch statements are like strategies that allow you to execute different code based on multiple conditions. For example:
var team = "Barcelona"; switch (team) { case "Barcelona": console.log("Visca el Barça!"); break; case "Real Madrid": console.log("Hala Madrid!"); break; default: console.log("Support your local team!"); break; }
In this example, we have a variable called team that has a value of “Barcelona“.
We use a switch statement to check the value of team and log a message to the console indicating which team we support.
Loops
Loops are like strategies that allow you to execute the same code multiple times. For example:
var players = ["Lionel Messi", "Cristiano Ronaldo", "Neymar Jr"]; for (var i = 0; i < players.length; i++) { console.log(players[i] + " is a great player!"); }
In this example, we have an array called players that contains three strings representing the names of players.
We use a for loop to iterate through the array and log a message to the console indicating that each player is a great player.
Functions
In football, players have different positions and roles, just like functions in JavaScript.
A function is like a player, and it has a name, position, and value. For example:
function shootBall(player) { console.log(player + " shoots the ball!"); } shootBall("Lionel Messi");
In this example, we have a function called shootBall that takes a parameter called player.
The function logs a message to the console indicating that the player shoots the ball.
We call the shootBall function and pass in “Lionel Messi” as the player parameter.
JavaScript also has anonymous functions, arrow functions, and higher-order functions that can take functions as parameters or return functions as values.
Learning how to use these advanced functions can take your JavaScript skills to the next level.
Conclusion
Understanding control flow and functions in JavaScript is like understanding tactics and strategies in football.
By mastering these concepts, you’ll be able to write more efficient, maintainable, and scalable code that can score some amazing goals!
Peace out!