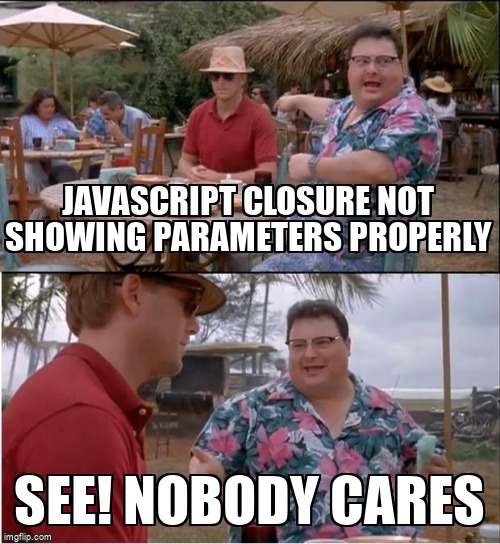
JavaScript: Closures and Generators
Hey there folks! Today, we’re going to talk about two powerful concepts in JavaScript: Closures and Generators.
Closures
In football, a striker is like a closure – they have access to the whole pitch and can use it to their advantage.
Similarly, closures in JavaScript have access to variables in the parent function, even after the parent function has returned. Let’s take an example:
function scorer(team) { var goals = 0; return function(name) { console.log(name + " scored a goal for " + team + "!"); goals++; console.log(team + " now has " + goals + " goals."); } } var score = scorer("Barcelona"); score("Lionel Messi"); score("Neymar Jr");
In this example, we define a function called scorer that takes a parameter called team.
The scorer function defines a variable called goals and returns an anonymous function that takes a parameter called name.
The anonymous function logs a message to the console indicating that a goal has been scored for the team, and updates the goals variable.
We create a variable called score that is assigned the return value of calling the scorer function with the argument “Barcelona“.
We call the score function twice, passing in “Lionel Messi” and “Neymar Jr” as arguments.
In this example, the anonymous function returned by scorer is a closure because it has access to the goals variable defined in the parent scorer function.
Each time we call the score function, the goals variable is updated and the new value is retained because of the closure.
Generators
In football, a midfielder is like a generator – they can control the pace of the game and create opportunities for the team.
Similarly, generators in JavaScript are functions that can pause and resume their execution, allowing for more efficient and flexible code. Let’s take an example:
function* midfielders() { yield "Lionel Messi"; yield "Andres Iniesta"; yield "Xavi Hernandez"; } var players = midfielders(); console.log(players.next().value); console.log(players.next().value); console.log(players.next().value);
In this example, we define a generator function called midfielders using the function* syntax.
The midfielders function uses the yield keyword to define a sequence of players.
We create a variable called players that is assigned the return value of calling the midfielders function.
We use the next method of the players object to iterate through the sequence of players, logging each player’s name to the console.
Conclusion
In conclusion, closures and generators are powerful concepts in JavaScript that can greatly improve your ability to write efficient and flexible code.
By understanding these concepts, you can take your JavaScript skills to the next level and score some amazing goals in your development projects.
Peace out!
Hi, cool website.