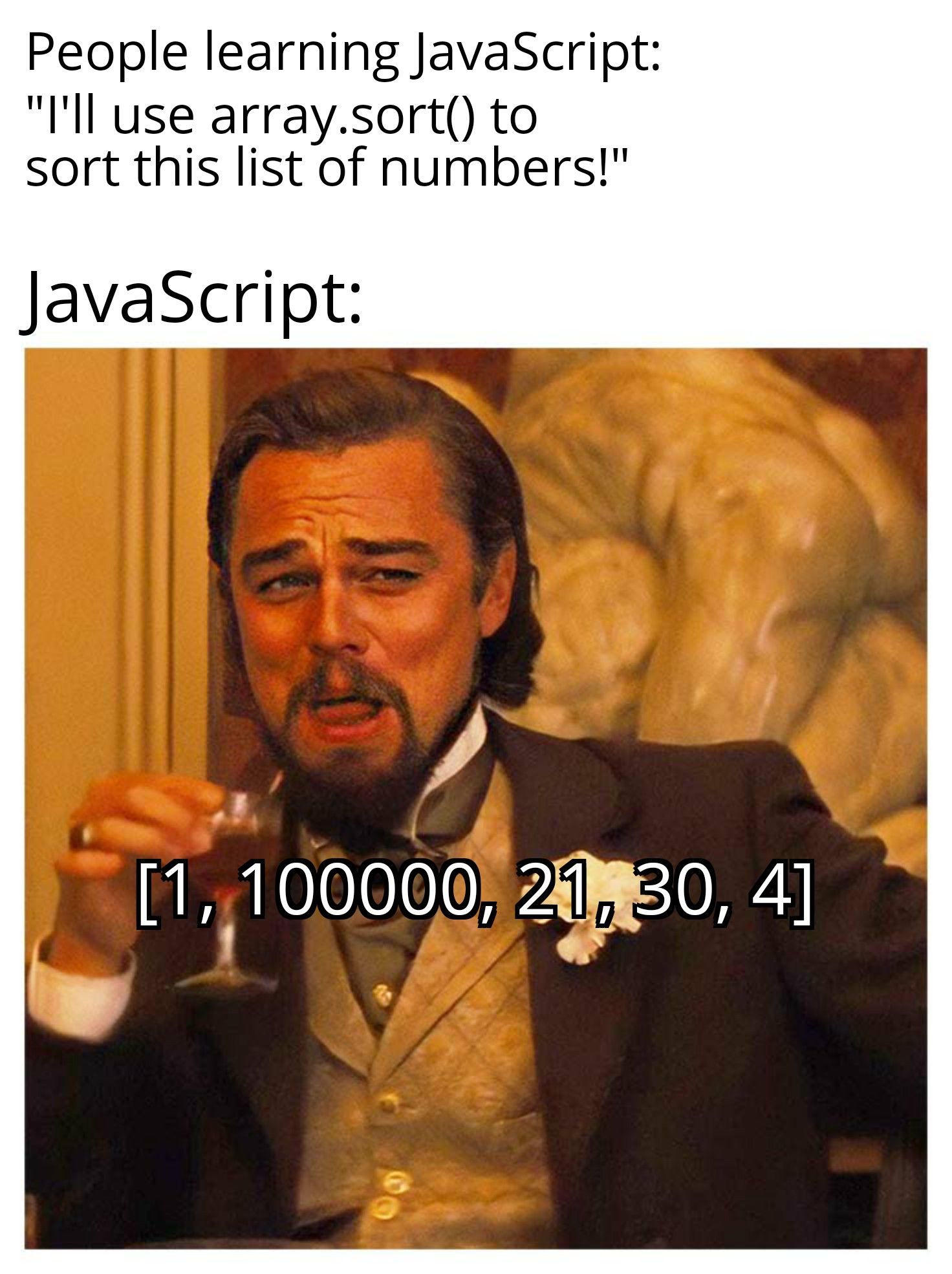
JavaScript Arrays and Objects
What’s up, football fans! Today, we’re gonna talk about JavaScript arrays and objects, the midfielders of the JavaScript game.
Arrays and objects are used to store and manipulate data in JavaScript, just like midfielders control the game and manipulate the ball.
Let’s dive into some examples using football terminology.
Arrays
In football, a team has a squad of players, just like an array in JavaScript.
An array is a collection of values, just like a list of players on a team. For example:
var players = ["Lionel Messi", "Cristiano Ronaldo", "Neymar Jr"]; console.log(players[0]); // outputs "Lionel Messi"
We can also use array methods to manipulate the contents of the array.
For example, we can add a new player to the end of the array using the push() method:
players.push("Mohamed Salah"); console.log(players); // outputs ["Lionel Messi", "Cristiano Ronaldo", "Neymar Jr", "Mohamed Salah"]
Objects
In football, a team has players with different positions and roles, just like objects in JavaScript.
An object is like a collection of variables and functions, bundled together. For example:
var player = { name: "Lionel Messi", position: "Forward", goals: 700 }; console.log(player.name); // outputs "Lionel Messi"
In this example, we have an object called player that has several properties, including name, position, and goals.
We can access individual properties of the object using dot notation.
We can also add or modify properties of the object using dot notation OR bracket notation
player.club = "Paris Saint-Germain"; player["goals"] = 703; console.log(player); // outputs {name: "Lionel Messi", position: "Forward", goals: 703, club: "Paris Saint-Germain"}
We can also use objects to represent more complex data structures, like a football match. For example:
var match = { homeTeam: "Barcelona", awayTeam: "Real Madrid", score: { home: 2, away: 1 }, referee: "Michael Oliver" }; console.log(match.score.home); // outputs 2 console.log(match["score"]["away"]); // outputs 1
In this example, we have an object called match that has several properties, including homeTeam, awayTeam, score, and referee.
The score property is itself an object with two properties, home and away.
We can access these nested properties using dot notation or bracket notation.
Conclusion
By using arrays and objects, we can represent and manipulate data in JavaScript just like midfielders control the game and manipulate the ball.
By mastering these concepts, you’ll be able to build powerful and complex applications with ease.
Peace out!