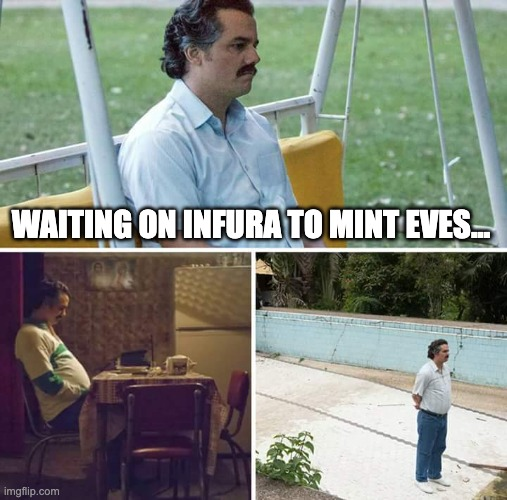
Infura
Yo, what’s up football fans? First things first, what is Infura?
Infura is a web3 API that allows you to interact with the Ethereum network without having to run your own Ethereum node.
This makes it super easy to build decentralized applications that rely on the Ethereum blockchain.
Let’s say you’re a Liverpool fan and you want to create an NFT that represents Liverpool’s historic 2019 UEFA Champions League win.
You could create a smart contract in Solidity that mints the NFT, but to interact with the Ethereum network, you’ll need to use a provider like Infura.
Here’s an example of how to use Infura with Solidity:
// import the Web3 library const Web3 = require('web3'); // import the contract ABI const contractABI = require('./contractABI.json'); // create a new instance of the Web3 library, and connect it to the Infura mainnet const web3 = new Web3(new Web3.providers.HttpProvider('https://mainnet.infura.io/v3/YOUR_PROJECT_ID')); // specify the contract address and create a new instance of the contract const contractAddress = '0x1234567890123456789012345678901234567890'; const contractInstance = new web3.eth.Contract(contractABI, contractAddress); // call the mintNFT function on the contract, passing in the required arguments // and specifying the gas limit and the wallet address to use for the transaction contractInstance.methods.mintNFT('Liverpool FC 2019 Champions League Winners', 'https://liverpoolfc.com/nft').send({ from: 'YOUR_WALLET_ADDRESS', gas: 500000 }) .then((receipt) => { console.log(receipt); // log the transaction receipt to the console });
In this example, we’re creating a new instance of the Web3 library and passing in the Infura provider URL and our project ID.
We’re also importing the contract ABI, which is a JSON file that defines the interface for our smart contract.
We’re then defining the contract address and creating a new instance of the contract using the contract ABI and address.
Finally, we’re calling the mintNFT function on the contract instance, passing in the NFT name and URI, as well as our wallet address and gas limit.
Once the transaction is sent, we can log the receipt to the console and verify that the NFT has been minted.
Conclusion
And there you have it, folks. Using Infura with Solidity is a great way to build decentralized applications that rely on the Ethereum blockchain.
And if you’re a Liverpool fan, you can create unique NFTs that represent the club’s historic moments.
So, whether you’re a football fan or not, consider exploring the world of Solidity and Infura and see what unique applications you can build.
Peace out!