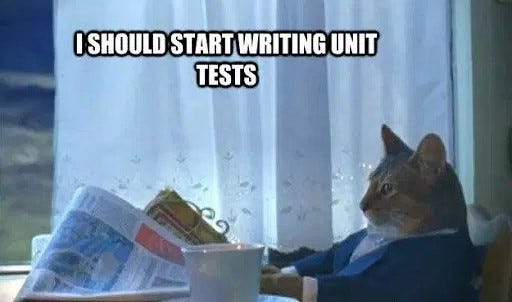
What’s up, football fans? Are you into Ethereum and smart contract development?
Then, you might want to learn how to Test your smart contracts using Chai and Hardhat.
Today, we’ll dive into the world of Solidity testing, and of course, we’ll use some Liverpool FC examples to spice things up.
Solidity a programming language that is used to write smart contracts on the Ethereum blockchain.
Smart contracts are self-executing contracts with the terms of the agreement between buyer and seller being directly written into lines of code.
Chai
Now, let’s talk about testing.
Testing is crucial to ensure that your smart contracts are working as intended.
It also ensure there are no vulnerabilities that could lead to potential attacks.
Chai is a popular assertion library that allows you to write human-readable tests,
Hardhat
Hardhat is a development environment that provides a lot of useful tools for testing and deploying your contracts.
Here are the steps to test your Solidity smart contracts with Chai and Hardhat:
Install Hardhat and Chai
The first step is to install Hardhat and Chai.
You can do this using npm by running the following commands:
npm install --save-dev hardhat chai @nomiclabs/hardhat-waffle
Create a test file
Next, you need to create a test file.
In Hardhat, test files should be placed in the test directory and should have the .js extension.
Here’s an example test file for a simple ERC20 token:
// Importing the necessary dependencies const { expect } = require('chai'); // Defining a test suite for MyToken smart contract describe('MyToken', function () { let myToken; // Creating an instance of MyToken smart contract before each test case beforeEach(async function () { const MyToken = await ethers.getContractFactory('MyToken'); myToken = await MyToken.deploy(); await myToken.deployed(); }); // Testing the total supply of MyToken smart contract it('should have a total supply of 1000000', async function () { const totalSupply = await myToken.totalSupply(); expect(totalSupply).to.equal(1000000); }); });
This test file creates a new ERC20 token, deploys it, and tests that the total supply is equal to 1000000.
Run the tests
Finally, you need to run the tests.
You can do this using the following command:
npx hardhat test
This will run all the test files in the test directory.
Now, let’s take a look at some examples.
Let’s say you’re building a betting platform for Liverpool FC matches, and you want to test your smart contract that handles bets.
Here’s an example test file using Chai and Hardhat:
const { expect } = require('chai'); // Tests for LiverpoolBetting contract describe('LiverpoolBetting', function () { let liverpoolBetting; // Deploy LiverpoolBetting contract before each test beforeEach(async function () { const LiverpoolBetting = await ethers.getContractFactory('LiverpoolBetting'); liverpoolBetting = await LiverpoolBetting.deploy(); await liverpoolBetting.deployed(); }); // Test that users can place bets it('should allow users to place bets', async function () { const userAddress = '0x1234567890123456789012345678901234567890'; const betAmount = ethers.utils.parseEther('1'); await liverpoolBetting.connect(signer).placeBet(userAddress, betAmount); const bet = await liverpoolBetting.bets(userAddress); expect(bet.amount).to.equal(betAmount); expect(bet.team).to.equal('Liverpool'); }); });
This test file creates a new instance of the LiverpoolBetting contract, deploys it, and tests that users can place bets on Liverpool FC.
It also checks that the bet amount and team are set correctly.
And that’s it! With these simple steps, you can test your Solidity smart contracts using Chai and Hardhat.
If you’re a Liverpool FC fan like me, you might be interested in building smart contracts related to football.
For example, you could create a token that represents ownership of a Liverpool FC match ticket.
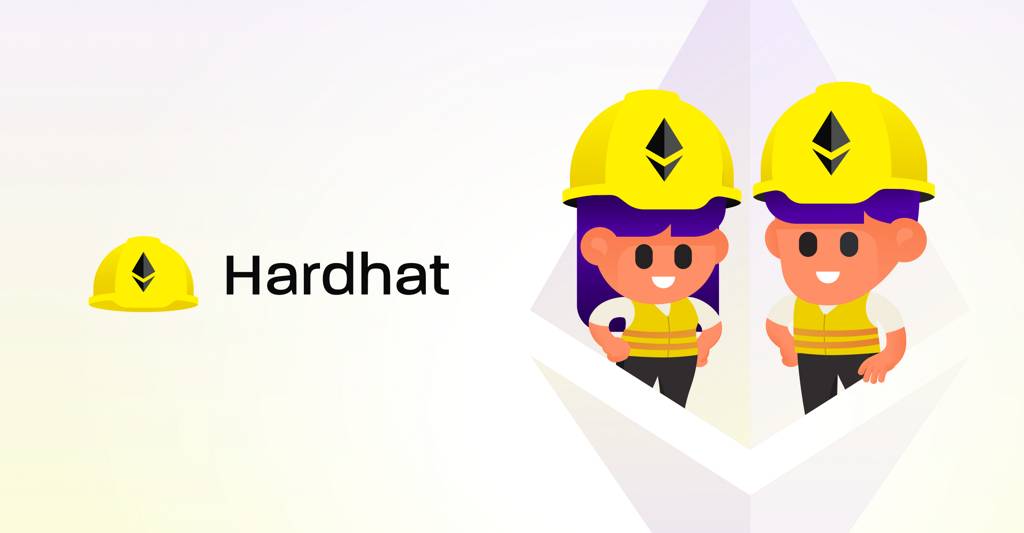
Conclusion
In conclusion, testing your Solidity smart contracts is crucial to ensure that they work correctly and that there are no security vulnerabilities.
Using tools like Chai and Hardhat can make testing easier and more efficient.
And if you’re a Liverpool FC fan, you can use your knowledge of Solidity to build some cool football-related smart contracts.
Good luck, and YNWA!
Peace out!