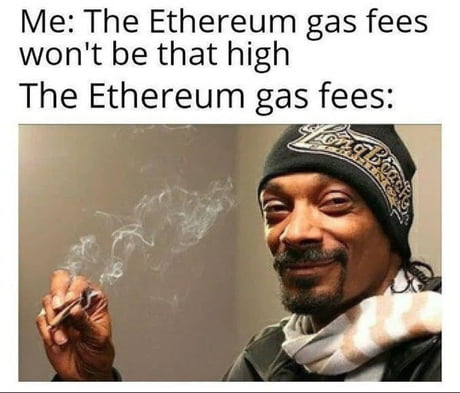
Gas Optimization
Hey, what’s up, football fans? Are you into blockchain development and Solidity?
If so, you may have heard of gas optimization.
Gas is the fee paid to miners for processing transactions on the Ethereum network.
Optimizing gas usage can save you a lot of money.
And if you’re a Liverpool FC fan like me, you’ll love the examples I’ve got for you today.
Let’s start with the basics. In Solidity, there are several ways to optimize gas usage.
Here are a few techniques:
Use smaller data types
Solidity has several data types, and some of them use more gas than others.
For example, uint256 uses more gas than uint8.
So, if you don’t need to use a larger data type, go for the smaller one.
Now, let’s look at some Liverpool-related examples.
Let’s say you’re building a decentralized betting platform where users can bet on Liverpool FC matches.
Here’s an example of how you can optimize gas usage by using smaller data types:
// Contract to update the score of a Liverpool match pragma solidity ^0.8.0; contract LiverpoolMatch { // Public variables to store the scores of Liverpool and their opponent uint8 public liverpoolScore; uint8 public opponentScore; // Function to update the score of a Liverpool match function updateScore(uint8 _liverpoolScore, uint8 _opponentScore) public { liverpoolScore = _liverpoolScore; opponentScore = _opponentScore; } }
In this example, we’re using uint8 for the liverpoolScore and opponentScore variables, which saves gas compared to using a larger data type like uint256.
Use constants
Solidity has a keyword called “constant” that you can use to define variables that never change.
Using constants can save gas because the compiler can replace the constant value directly in the bytecode.
Here’s an example of how you can use constants to save gas:
// Define constants for possible match outcomes uint constant LIVERPOOL_WIN = 1; uint constant OPPONENT_WIN = 2; uint constant DRAW = 3; // Function to get the result of the match based on the scores function getResult() public view returns (uint) { // Check if Liverpool won if (liverpoolScore > opponentScore) { return LIVERPOOL_WIN; } // Check if opponent won else if (opponentScore > liverpoolScore) { return OPPONENT_WIN; } // Otherwise, it's a draw else { return DRAW; } }
In this example, we’re using constants to define the possible outcomes of the match.
By doing this, we’re saving gas because the compiler can replace the constant values directly in the bytecode.
Avoid using loops
Loops can be gas-intensive, especially if they iterate over a large number of items.
Try to avoid using loops whenever possible.
Here’s an example of how you can avoid using loops to save gas:
// Define a mapping that associates an address with a bet amount mapping (address => uint) public bets; // Define a function that takes an array of addresses and returns an array of bet amounts function getBets(address[] memory _users) public view returns (uint[] memory) { uint[] memory userBets = new uint[](_users.length); for (uint i = 0; i < _users.length; i++) { userBets[i] = bets[_users[i]]; } return userBets; }
In this example, we’re using a mapping to store the bets of each user.
And we’re using the getBets function to return an array of bets for a list of users.
We do this by using a mapping and returning an array in one function call,
We’re avoiding the need for a loop, which saves gas.
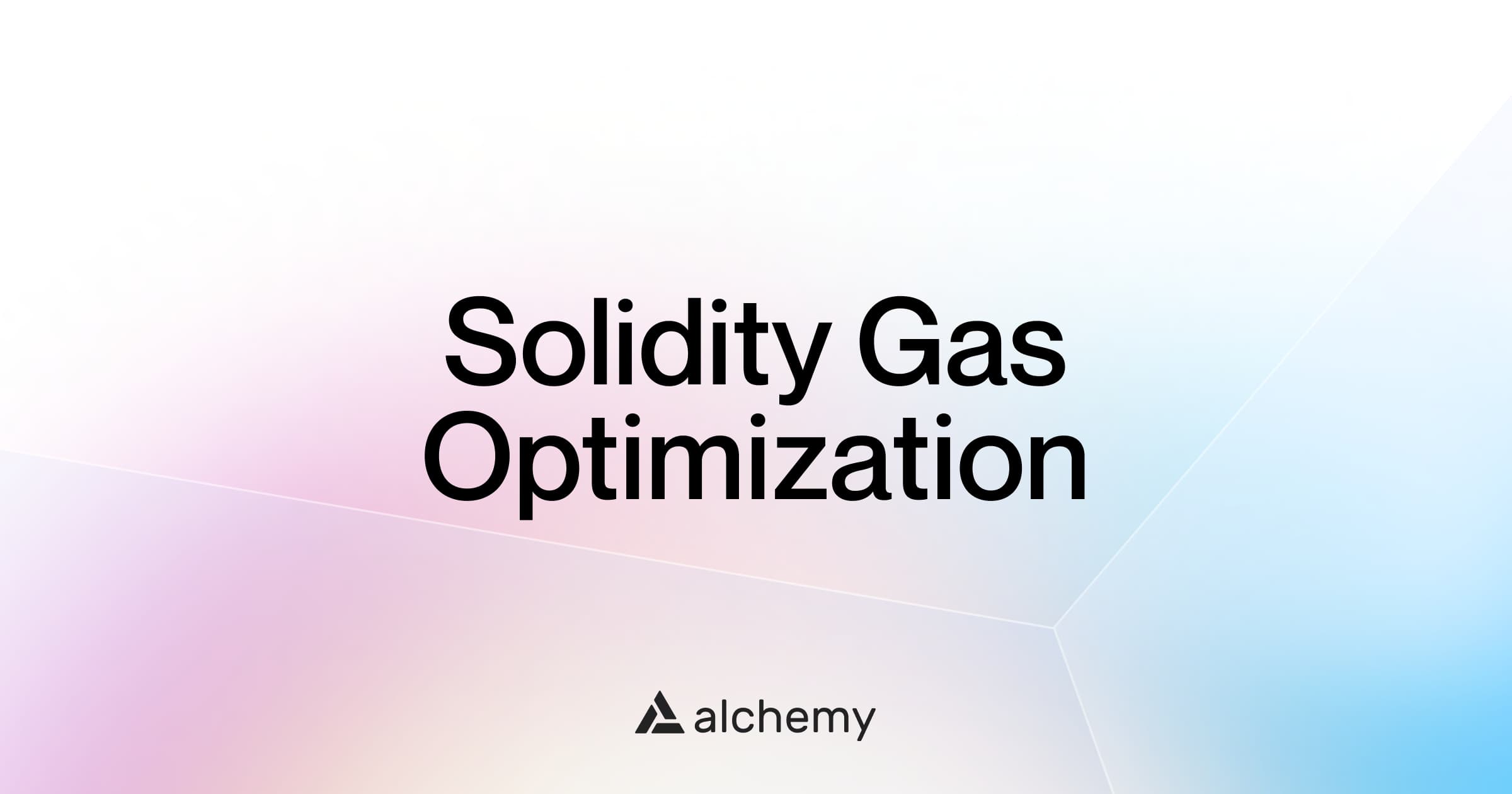
Conclusion
So, there you have it, folks.
That’s how you can optimize gas usage in Solidity. By using smaller data types, constants, and avoiding loops, you can save gas and reduce the cost of your transactions.
And if you’re a Liverpool fan, you can apply these techniques to your own blockchain projects, whether you’re building a decentralized betting platform or a fan token for Liverpool FC.
Peace out!