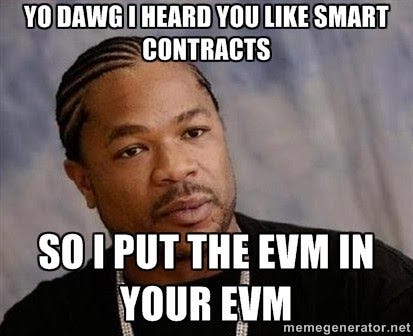
Ethereum Virtual Machine (EVM)
Hey football fans, today we are gonna explore EVM’s. So What is the Ethereum Virtual Machine (EVM)?
The EVM is a virtual machine that runs on the Ethereum blockchain.
It’s responsible for executing smart contracts written in Solidity, which are essentially self-executing contracts that enforce the rules of a particular application or transaction.
Smart contracts can be used for a variety of purposes, including financial transactions, voting systems, and supply chain management.
The EVM is similar to a traditional computer in that it has its own memory, storage, and processing power.
However, unlike a traditional computer, the EVM is decentralized, meaning that it’s not controlled by a single entity or organization.
Instead, it’s run by nodes on the Ethereum network, which are distributed around the world.
Let’s say that Liverpool FC wants to create a smart contract to manage their merchandise sales using the Ethereum Virtual Machine (EVM).
Here’s an example of what the Solidity code for this smart contract might look like:
pragma solidity ^0.8.0; // Define the smart contract contract LiverpoolMerchandise { // Define a public variable for the price of the merchandise uint public price; // Define a mapping that associates addresses with the amount of ether sent to the contract mapping(address => uint) public balances; // Define a constructor function that sets the initial price of the merchandise constructor(uint _price) { price = _price; } // Define a function for fans to buy merchandise by sending ether to the contract function buyMerchandise() public payable { // Require that the amount of ether sent is greater than or equal to the price of the merchandise require(msg.value >= price, "Insufficient funds"); // Add the amount of ether sent to the buyer's balance in the mapping balances[msg.sender] += msg.value; } // Define a function for the contract owner to withdraw funds from the contract function withdrawFunds() public { // Require that the caller has a positive balance in the mapping require(balances[msg.sender] > 0, "No funds to withdraw"); // Transfer the balance from the mapping to the caller's address payable(msg.sender).transfer(balances[msg.sender]); // Set the caller's balance in the mapping to 0 balances[msg.sender] = 0; } }
In this example, we’re creating a smart contract called “LiverpoolMerchandise” that has a public variable for the price of the merchandise
And a mapping that associates addresses with the amount of ether that has been sent to the contract.
The contract also has two functions: “buyMerchandise” and “withdrawFunds“.
The buyMerchandise function is used to buy merchandise by sending ether to the contract, while the withdrawFunds function is used to withdraw funds from the contract.
Once this code is written, it can be compiled using a Solidity compiler, which generates bytecode that can be executed by the EVM.
The bytecode is then uploaded to the Ethereum network, where it can be executed by nodes on the network.
With this smart contract, Liverpool FC can easily sell merchandise to their fans using the security and transparency of the blockchain.

Conclusion
In conclusion, the EVM is a critical component of the Ethereum blockchain, as it’s responsible for executing smart contracts written in Solidity.
So, if you’re interested in blockchain development or just want to learn a new programming language, EVM is definitely worth exploring.
And if you’re a Liverpool FC fan, you can even use your knowledge of blockchain to create smart contracts for managing ticket sales, merchandise sales, and more.
Who knows, maybe one day Liverpool FC will be one of the first football clubs to adopt blockchain technology in a big way!
Peace out!