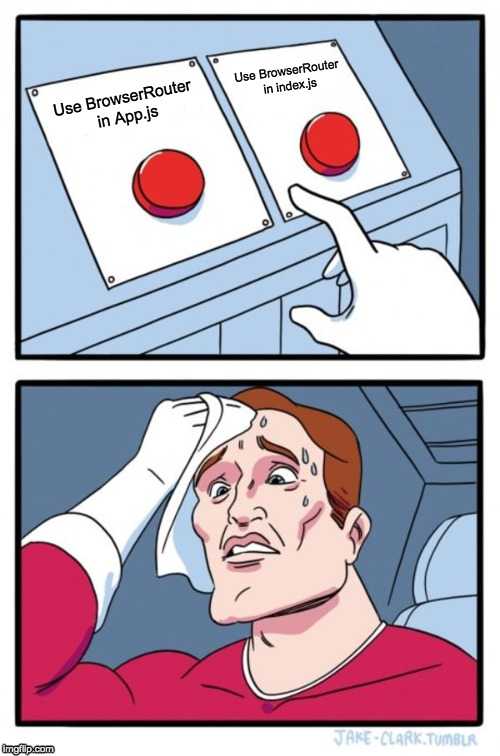
Hey, what’s up Liverpool FC fans! Are you ready to take your React app to the next level? Then you gotta learn about React Router, a routing library for React.
Here, we’ll talk about what React Router is and how to use it with some dope examples.
React Router
So, what is React Router? Simply put, it’s a library that allows you to handle navigation and routing within your React application.
With React Router, you can create different routes for different components and URLs.
This means that when a user clicks on a link, they’ll be taken to a different page without the page refreshing.
Let’s dive into some examples using Liverpool-related terminology.
Imagine you’re building an app that displays information about Liverpool FC players.
You want to create different routes for each player’s information, so you can display their stats, bio, and photos.
Here’s how you can use React Router to do that
Install React Router
First, you need to install React Router. You can do this via npm by running the command “npm install react-router-dom“.
Set up your routes
Next, you need to set up your routes. You can do this by creating a new file called “routers.js” and adding the following code:
import { BrowserRouter as Router, Routes, Route } from "react-router-dom"; import PlayerPage from "./PlayerPage"; // Define a function component called Routes function Routers() { // Render a Router component with a Switch component as its child return ( <Router> <Routes> {/* Render a Route component with a path prop that includes a URL parameter for the player name, and a component prop that references the PlayerPage component */} <Route path="/players/:playerName" component={PlayerPage} /> </Routes> </Router> ); } // Export the Routes component as the default export of the module export default Routes;
In this code, we’re importing the necessary components from React Router, and creating a new route for each player’s page.
The “:playerName” is a dynamic parameter that will be replaced with the name of the player in the URL.
Create your player page component
Now it’s time to create the component that will be rendered when a user navigates to a player’s page.
Here’s an example
import React from "react"; import { useParams } from "react-router-dom"; // Define a function component called PlayerPage function PlayerPage() { const { playerName } = useParams(); // Get the player name URL parameter using the useParams hook // Render a component that displays the player name and a placeholder message for stats, bio, and photos return ( <div> <h1>{playerName}</h1> <p>Stats, bio, and photos for {playerName} go here.</p> </div> ); } // Export the PlayerPage component as the default export of the module export default PlayerPage;
In this code, we’re using the “useParams” hook from React Router to get the value of the dynamic parameter in the URL.
We’re then rendering the player’s name and some placeholder text
Add links to your app
Finally, you need to add links to your app so that users can navigate to the different player pages.
Here’s an example
import React from "react"; import { Link } from "react-router-dom"; // Define a function component called PlayerList function PlayerList() { // Render a list of players with links to their respective PlayerPage component return ( <div> <ul> <li> <Link to="/players/mo-salah">Mo Salah</Link> {/* Link to the Mo Salah player page */} </li> <li> <Link to="/players/sadio-mane">Sadio Mane</Link> {/* Link to the Sadio Mane player page */} </li> <li> <Link to="/players/virgil-van-dijk">Virgil van Dijk</Link> {/* Link to the Virgil van Dijk player page */} </li> </ul> </div> ); } // Export the PlayerList component as the default export of the module export default PlayerList;

Conclusion
In this code, we’re using the “Link” component from React Router to create links to each player’s page.
When a user clicks on a link, they’ll be taken to the corresponding player page without the page refreshing.
And that’s it! With React Router, you can easily handle navigation and routing within your React application.
Peace out!