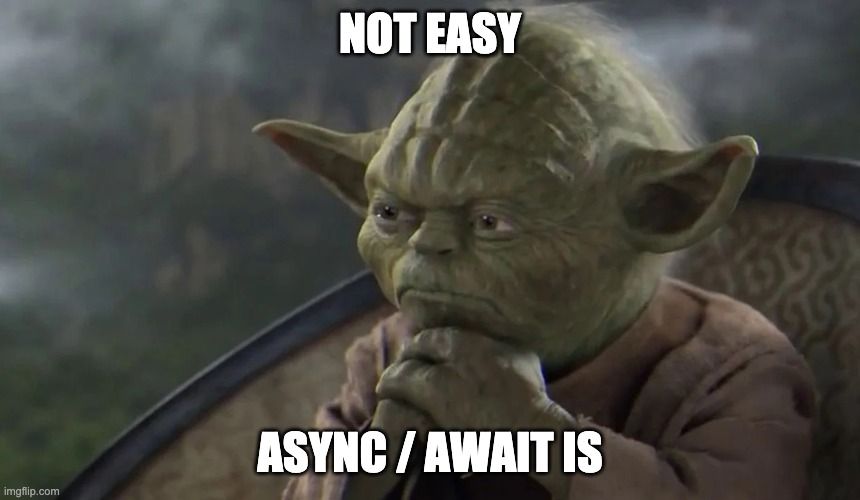
Javascript Promises
Promises are like football promises – you might not know if they’ll come through until the end of the game.
In JavaScript, Promises handle asynchronous code that may or may not complete successfully.
They represent a value that may be available now, in the future, or never.
Here’s an example using football lingo:
function scoreGoal() { return new Promise((resolve, reject) => { setTimeout(() => { const score = Math.floor(Math.random() * 10); if (score > 5) { resolve(score); } else { reject("Failed to score!"); } }, 1000); }); }
In this example, we have a function called scoreGoal that returns a new Promise.
The Promise resolves with a random score between 0 and 10 after 1 second.
If the score > 5, the Promise resolves with the score.
Otherwise, the Promise rejects with an error message.
JavaScript Async / Await
Async/Await, on the other hand, is like waiting for the referee to blow the whistle.
It can be frustrating, but it’s necessary to keep the game fair.
In JavaScript, Async/Await makes it easier to write and read asynchronous code that behaves like synchronous code.
Here’s an example using football terminology:
async function playMatch() { console.log("Match started!"); await wait(2000); console.log("Half time!"); await wait(2000); console.log("Second half started!"); await wait(2000); console.log("Match ended!"); }
In this example, we have an async function called playMatch that logs messages to the console at different points during a match.
The await keyword is used to wait for a Promise to resolve before continuing with the next line of code.
This allows the code to behave synchronously, even though it’s running asynchronously.
Conclusion
By using Promises and Async/Await in your JavaScript code, you can improve the performance and responsiveness of your applications.
So next time you’re waiting for your code to finish running, just think of it as a football match – you might not know the outcome until the end, but with Promises and Async/Await, you’ll get there faster and with fewer errors.
Peace out!