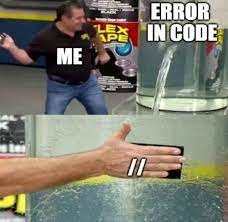
Error Handling in JavaScript
Yo, what’s up football fans! Today, we’re going to talk about error handling in JavaScript. Just like in football, errors can happen in your code, but with the right techniques, you can keep your application running smoothly. So let’s get started!
In JavaScript, an error is an unexpected condition that prevents your code from executing properly.
Some common types of errors include syntax errors, runtime errors, and logic errors.
Error handling is the process of detecting and managing errors in your code.
Types of Errors:
Syntax Errors: Syntax errors occur when there is a mistake in your code’s syntax.
For example, if you forget to close a bracket or misspell a keyword, the code will not be able to run.
Runtime Errors: Runtime errors occur when your code is running and encounters an unexpected situation. For example, if you try to divide a number by zero, an error will occur because it’s not a valid operation.
Logic Errors: Logic errors occur when your code executes, but the output is not what you expected.
For example, if you write a function to calculate the average of two numbers, but forget to divide by two, the result will be incorrect.
Try-Catch Statement:
One of the most common ways to handle errors in JavaScript is by using a try-catch statement.
The try block contains the code that might throw an error, and the catch block contains the code that handles the error. For example:
try { // code that might throw an error } catch (error) { // code that handles the error }
In football terms, it’s like a goalkeeper trying to save a goal (the try block), and if the ball goes past him, the defender tries to clear the ball (the catch block).
Here’s an example of using try-catch to handle a runtime error:
try { var result = 10 / 0; console.log(result); } catch (error) { console.log("An error occurred: " + error.message); }
In this example, we try to divide 10 by 0, which is not a valid operation.
This will throw a runtime error, which we catch in the catch block.
We log a message to the console indicating that an error occurred and display the error message.
Throw Statement
Another way to handle errors in JavaScript is by using the throw statement.
The throw statement allows you to create a custom error object and throw it when an error occurs. For example:
function calculateAverage(num1, num2) { if (typeof num1 !== "number" || typeof num2 !== "number") { throw new Error("Both arguments must be numbers."); } return (num1 + num2) / 2; } try { var result = calculateAverage(10, "20"); console.log(result); } catch (error) { console.log("An error occurred: " + error.message); }
In this example, we define a function called calculateAverage that takes two arguments.
If either argument is not a number, we throw a new error object with a custom message.
We use a try-catch statement to handle the error and log a message to the console.
Finally Block
The finally block is used to execute code after a try-catch block, regardless of whether an error occurred or not.
This can be useful for cleaning up resources, such as closing a database connection or releasing memory. For example:
try { // code that might throw an error } catch (error) { // code that handles the error } finally { // code that is always executed }
In football terms, it’s like a post-match interview with the coach and players (the finally block) where they discuss the game, win or lose.
Here’s an example of using the finally block:
try { console.log("Try block"); throw new Error("Error occurred"); } catch (error) { console.log("Catch block: " + error.message); } finally { console.log("Finally block"); }
In this example, we have a try block that logs a message to the console and throws a new error.
The catch block logs a message that displays the error message.
The finally block logs a message to the console, which will always be executed, regardless of whether an error occurred or not.
Conclusion
In conclusion, error handling is an essential part of JavaScript programming.
By using techniques such as try-catch, throw, and finally blocks, you can detect and manage errors in your code effectively.
Just like in football, being able to handle errors and unexpected situations can greatly improve your performance and lead to success.
Peace out!