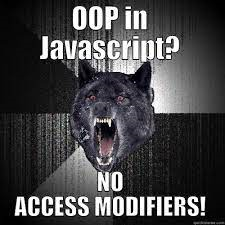
Regular Expressions
Hey there, folks! Today, we’re gonna talk about Regular Expressions in JavaScript.
Regular expressions are like the goalkeepers of JavaScript. They can catch anything that matches a pattern and give you control over your data.
So, let’s dive into some JavaScript Regular Expression examples using football terminology.
Basic Syntax
In football, teams have different formations, just like Regular Expressions in JavaScript.
A Regular Expression is like a pattern that you want to match. For example:
var pattern = /Messi/;
In this example, we have a Regular Expression called pattern that matches the string “Messi“.
The forward slash / at the beginning and end of the expression indicate that this is a Regular Expression.
Matchin Strings
In football, you can match players to their positions, just like you can match strings to Regular Expressions in JavaScript. For example:
var player = "Lionel Messi"; var position = /Messi/; if (player.match(position)) { console.log("Messi is a forward!"); }
In this example, we have a string called player that contains the name “Lionel Messi”.
We also have a Regular Expression called position that matches the string “Messi”.
We use the match method to check if the player string matches the position Regular Expression.
If there’s a match, we log a message to the console indicating that Messi is a forward.
Modifiers
In football, players have different skills and abilities, just like Regular Expressions have modifiers in JavaScript.
Modifiers are like flags that change how Regular Expressions match strings. For example:
var player = "Lionel Messi"; var position = /messi/i; if (player.match(position)) { console.log("Messi is a forward!"); }
In this example, we have a Regular Expression called position that matches the string “messi” using the i modifier.
The i modifier makes the Regular Expression case-insensitive, so it matches both “Messi” and “messi”.
Character Classes
In football, players wear different jersey numbers, just like Regular Expressions can match different characters using character classes in JavaScript.
Character classes are like sets of characters that match a specific pattern. For example:
var player = "Lionel Messi"; var jerseyNumber = /[0-9]/; if (player.match(jerseyNumber)) { console.log("Messi wears a numbered jersey!"); }
In this example, we have a Regular Expression called jerseyNumber that matches any single digit.
We use the match method to check if the player string contains a digit.
If there’s a match, we log a message to the console indicating that Messi wears a numbered jersey.
Quantifiers
In football, teams have different formations and number of players on the field, just like Regular Expressions can match a specific number of characters using quantifiers in JavaScript.
Quantifiers are like modifiers that specify how many times a character should be matched. For example:
var player = "Lionel Messi"; var position = /Messi{2}/; if (player.match(position)) { console.log("Messi is a forward!"); }
In this example, we have a Regular Expression called position that matches the string “Messi” exactly two times.
We use the match method to check if the player string matches the position Regular Expression.
If there’s a match, we log a message to the console indicating that Messi is a forward.
By using Regular Expressions in JavaScript, you can match patterns in strings, validate user input, and manipulate text data in powerful ways.
These examples are just the tip of the iceberg when it comes to using Regular Expressions in JavaScript.
Conclusion
In conclusion, Regular Expressions are a powerful tool for manipulating and validating text data in JavaScript.
By using Regular Expressions, you can match patterns, validate user input, and manipulate text data in powerful ways.
So, whether you’re a football fan or not, Regular Expressions are an essential tool for any JavaScript developer.
Peace out!