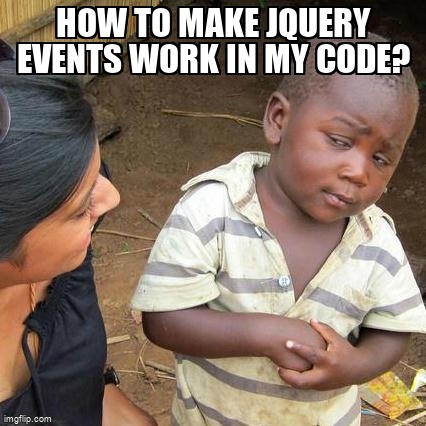
Yo, what’s good, my homies? Today, we’re gonna talk about event handling with jQuery.
This is like the base for creating some dope-a$$ web pages that are interactive and lit, ya feel me?
jQuery makes it easy to handle events, and we’re gonna explore some of the basics.
Event Binding
First off, we got event binding.
Event Binding is like when you attach an event handler function to an HTML element.
jQuery got mad methods for event binding, like .click(), .hover(), and .submit(). Check it:
// Attach a click event handler to a button element $('#my-button').click(function() { alert('Button clicked!'); });
In this example, we’re using the .click() method to attach a click event handler to a button element with an ID of my-button.
When the button is clicked, the anonymous function will be executed, which displays an alert box with the message “Button clicked!”.
Event Delegation
Event Delegation is like when you attach an event handler to a parent element that handles events triggered by its child elements.
This is real handy when you’re dealing with content that’s generated dynamically. Check it:
// Attach a click event handler to a parent element that handles clicks on its child elements $('#my-parent').on('click', '.my-child', function() { alert('Child element clicked!'); });
In this example, we’re attaching a click event handler to a parent element with an ID of my-parent.
The second argument to the .on() method is a selector for the child elements we want to target, in this case, elements with a class of my-child.
When a child element is clicked, the anonymous function will be executed, which displays an alert box with the message “Child element clicked!”.
jQuery got a whole bunch of events that you can use to make your web pages fly
Some of the most common events include:
click: This is like when you click something, ya dig?
hover: This is like when you hover over something with your mouse, like a boss.
submit: This is like when you submit a form, no cap.
keydown: This is like when you press a key down on your keyboard, like a G.
keyup: This is like when you release a key on your keyboard, like a playa.
Here’s an example that demonstrates the use of the click event:
// Attach a click event handler to a button element $('#my-button').click(function() { // Toggle the visibility of a div element $('#my-div').toggle(); });
In this example, we’re attaching a click event handler to a button element with an ID of my-button.
When the button is clicked, the .toggle() method is called on a div element with an ID of my-div, which toggles its visibility.
Conclusion
jQuery event handling is a must-have skill if you’re into web development.
By using event binding and event delegation, you can create some sick web pages that respond to user actions and provide a dope user experience.
So, go ahead and experiment with these concepts, and make your web pages pop!
Peace out!